Interact with Users
Visual Basic provides two simple ways to interact with users:
Use
InputBox
to get text input.Use
MsgBox
to display short messages and get button-click responses.
I’ve already used InputBox
and MsgBox
a number of times in previous examples, but one more sample won’t hurt:
Sub MsgBoxInputBox( ) Dim str As String, val As VbMsgBoxResult ' InputBox gets simple text input. str = InputBox("Enter some text.", "Chapter 3", "Some text") ' Use If to test if a value was entered. If str <> "" Then ' You can combine style constants in MsgBox. val = MsgBox(str, vbQuestion + vbOKCancel, "Chapter 3") ' Return value indicates which button was clicked. If val = vbOK Then Debug.Print "OK" Else Debug.Print "Cancel" End If End Sub
The preceding code displays a simple dialog box to get text, then displays the text in another simple dialog box, as shown in Figure 3-1.
The MsgBox
function can display many different styles and buttons, depending on the Button
argument setting. All of the VbMsgBoxStyle
settings are listed in Table 3-2.
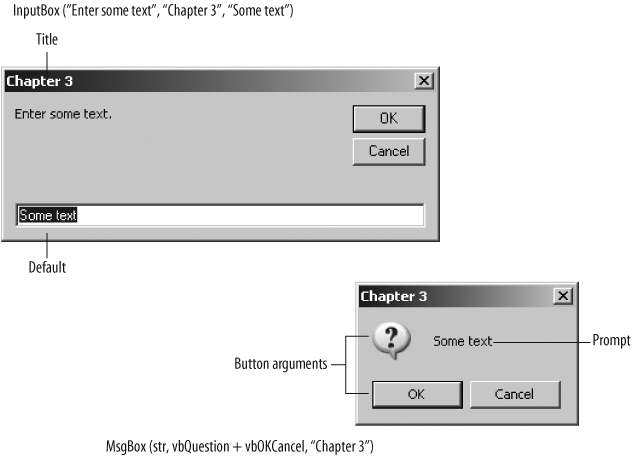
Figure 3-1. InputBox and MsgBox functions display simple dialog boxes
Table 3-2. VbMsgBoxStyle settings
Setting |
Description |
---|---|
Button | |
|
Displays OK button. |
|
Displays OK, Cancel buttons. |
|
Displays Abort, Retry, Ignore buttons. |
|
Displays Yes, No, Cancel buttons. |
|
Displays Yes, No buttons. ... |
Get Programming Excel with VBA and .NET now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.