Moving an Image
Moving an image on a cubic Bezier curve path is just as easy as moving a circular drawing object, as we’ll demonstrate in the next two examples. Suppose you are making a game where bull’s-eyes move across the canvas and the player must shoot at them. You could use cubic Bezier curve paths to create new and interesting patterns for the bull’s-eyes to move along.
For this example, we first create a global variable named bullseye
, which we will use to hold the
bullseye.png image that we will
load to display on the canvas:
var
bullseye
;
function
eventWindowLoaded
()
{
bullseye
=
new
Image
();
bullseye
.
src
=
"bullseye.png"
bullseye
.
onload
=
eventAssetsLoaded
;
}
In canvasApp()
, we will create
a different path for the curve from the one in the first example by
setting new values for p0
, p1
, p2
, and
p3
. Using these values, the bullseye
will move on a parabola-like path.
(Figure 5-16 shows the
path of the curve.)
var
p0
=
{
x
:
60
,
y
:
10
};
var
p1
=
{
x
:
150
,
y
:
350
};
var
p2
=
{
x
:
300
,
y
:
375
};
var
p3
=
{
x
:
400
,
y
:
20
};
We also need to create a player
object that represents the bull’s-eye on the canvas:
var
player
=
{
x
:
0
,
y
:
0
,
speed
:
.
01
,
t
:
0
};
In drawImage()
, after we
calculate t
, xt
, and yt
,
we draw the image on the canvas:
player
.
x
=
xt
-
bullseye
.
width
/
2
;
player
.
y
=
yt
-
bullseye
.
height
/
2
;
context
.
drawImage
(
bullseye
,
player
.
x
,
player
.
y
);
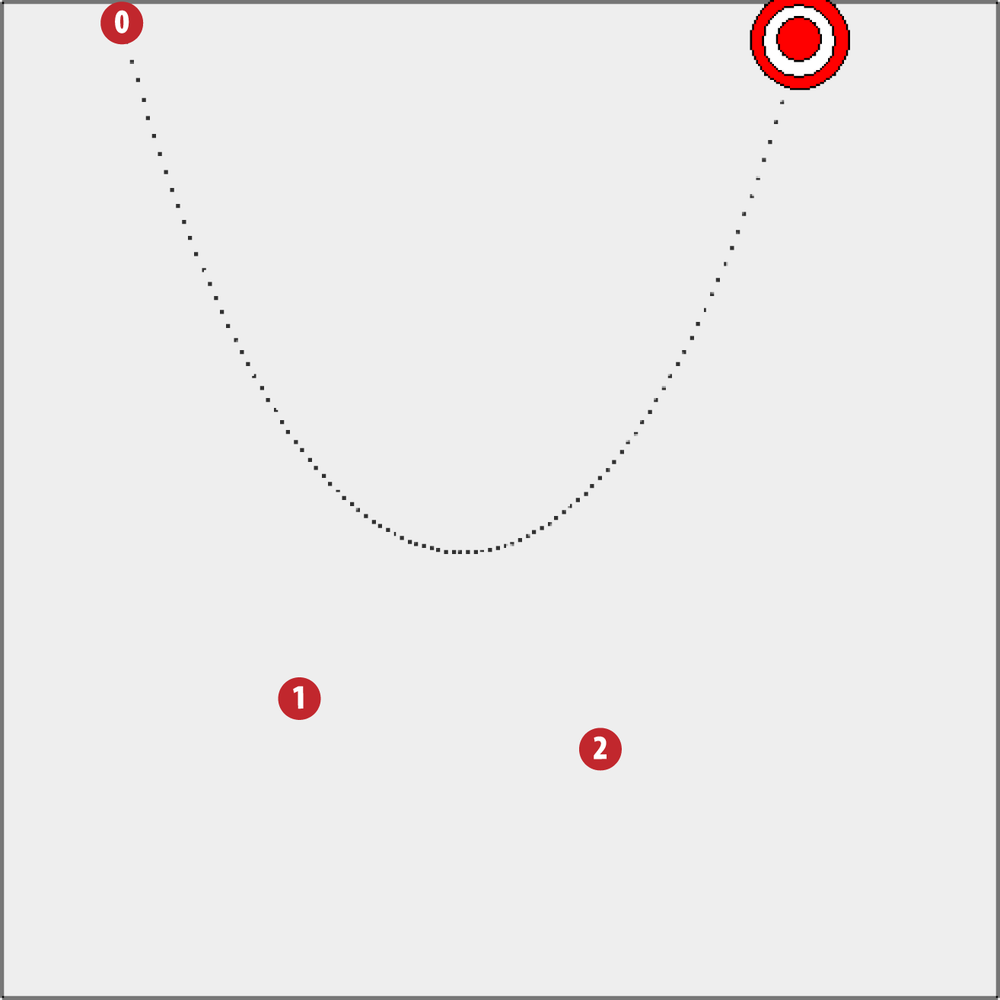
Figure 5-16. Moving an image on a cubic Bezier curve path
The rest ...
Get HTML5 Canvas, 2nd Edition now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.