UIImage Drawing
A UIImage provides methods for drawing itself into the current context. We now know how to obtain an image context and make it the current context, so we can experiment with these methods. Here, I’ll make a UIImage consisting of two pictures of Mars side by side:
UIImage* mars = [UIImage imageNamed:@"Mars.png"]; CGSize sz = [mars size]; UIGraphicsBeginImageContextWithOptions( CGSizeMake(sz.width*2, sz.height), NO, 0); [mars drawAtPoint:CGPointMake(0,0)]; [mars drawAtPoint:CGPointMake(sz.width,0)]; UIImage* im = UIGraphicsGetImageFromCurrentImageContext(); UIGraphicsEndImageContext();
The resulting UIImage im
is suitable anywhere you would use a UIImage. For instance, you could hand it over to a visible UIImageView, thus causing the image to appear onscreen (Figure 15-6).
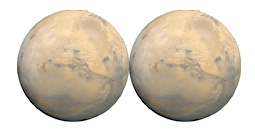
Additional UIImage methods let you scale an image into a desired rectangle as you draw, and specify the compositing (blend) mode whereby the image should combine with whatever is already present. To illustrate, I’ll create an image showing Mars centered in another image of Mars that’s twice as large, using the Multiply blend mode (Figure 15-7):
UIImage* mars = [UIImage imageNamed:@"Mars.png"]; CGSize sz = [mars size]; UIGraphicsBeginImageContextWithOptions( CGSizeMake(sz.width*2, sz.height*2), NO, 0); [mars drawInRect:CGRectMake(0,0,sz.width*2,sz.height*2)]; ...
Get Programming iOS 6, 3rd Edition now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.