Chapter 16. Classes and Functions
Now that we know how to create new types, the next step is to write functions that take programmer-defined objects as parameters and return them as results. In this chapter I also present “functional programming style” and two new program development plans.
Code examples from this chapter are available from https://thinkpython.com/code/Time1.py. Solutions to the exercises are at https://thinkpython.com/code/Time1_soln.py.
Time
As another example of a programmer-defined type, we’ll define a class called Time
that records the time of day. The class definition looks like this:
class Time: """Represents the time of day. attributes: hour, minute, second """
We can create a new Time
object and assign attributes for hours, minutes, and seconds:
time = Time() time.hour = 11 time.minute = 59 time.second = 30
The state diagram for the Time
object looks like Figure 16-1.
As an exercise, write a function called print_time
that takes a Time object and prints it in the form hour:minute:second
. Hint: the format sequence '%.2d'
prints an integer using at least two digits, including a leading zero if necessary.
Write a boolean function called is_after
that takes two Time objects, t1
and t2
, and returns True
if t1
follows t2
chronologically and False
otherwise. Challenge: don’t use an if
statement.
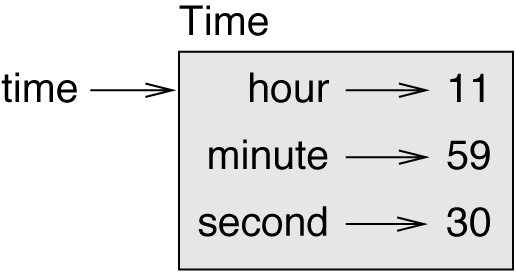
Figure 16-1. Object diagram.
Pure Functions
In the next few sections, we’ll ...
Get Think Python, 2nd Edition now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.