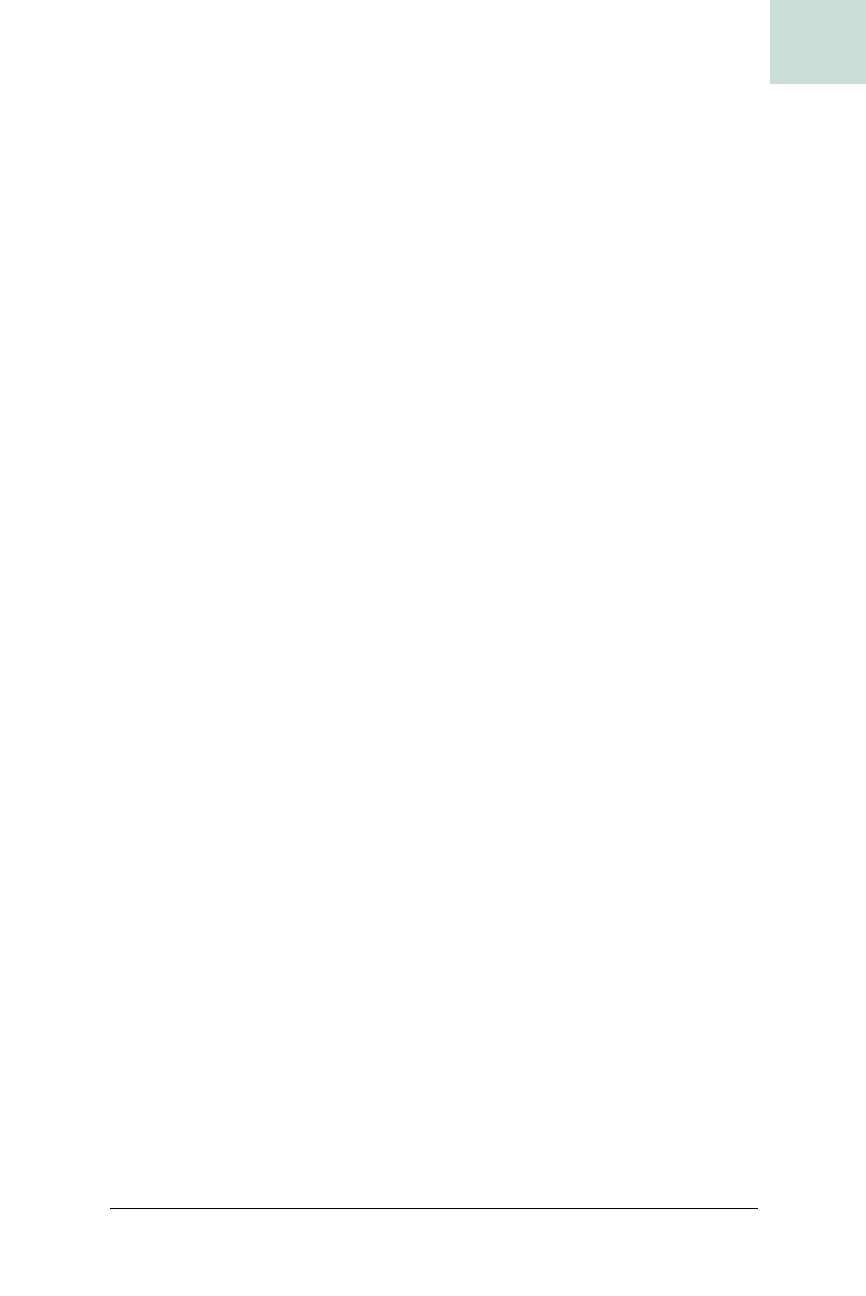
Search Through JTables Easily #26
Chapter 3, Tables and Trees
|
135
HACK
public int getRowCount( ) {
return (tableModel == null) ? 0 : rowToModelIndex.size( );
}
public boolean isCellEditable(int row, int column) {
return tableModel.isCellEditable(getModelRow(row), column);
}
public Object getValueAt(int row, int column) {
return tableModel.getValueAt(getModelRow(row), column);
}
public void setValueAt(Object aValue, int row, int column) {
tableModel.setValueAt(aValue, getModelRow(row), column);
}
Indexing
Lucene is a document indexing and searching tool, available from http://
lucene.apache.org/. To incorporate it into a Java application, you simply need
to put its JAR file, typically named something like lucene-
version.jar, into
your classpath. For this hack, you’ll need the following
import statements:
import org.apache.lucene.store.*;
import org.apache.lucene.document.*;
import org.apache.lucene.analysis.*;
import org.apache.lucene.index.*;
import org.apache.lucene.search.*;
import org.apache.lucene.queryParser.*;
Most developers use Lucene with documents, but you can fake it to index a
table model instead. First, you need to create a Lucene index, where all of
the internal Lucene links are stored. You should use a
RAMDirectory rather
than a file-based directory in order to keep everything portable. You’ll also
need an
Analyzer that helps communicate between data and the index, as
well as an
IndexWriter that actually writes to the index:
directory = new RAMDirectory( );
analyzer = new WhitespaceAnalyzer( );
IndexWriter writer = new IndexWriter(directory, analyzer, true);
For the purposes of indexing, think of every row as a document and every
column as a word or a set of words (called a
Field in Lucene-speak) for that
document. Now, loop through the table model’s rows, creating a new
Document for each row and add Fields per column:
for (int row=0; row < tableModel.getRowCount( ); row++){
Document document = new Document( );
//add fields
writer.addDocument(document);
}
Get Swing Hacks now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.