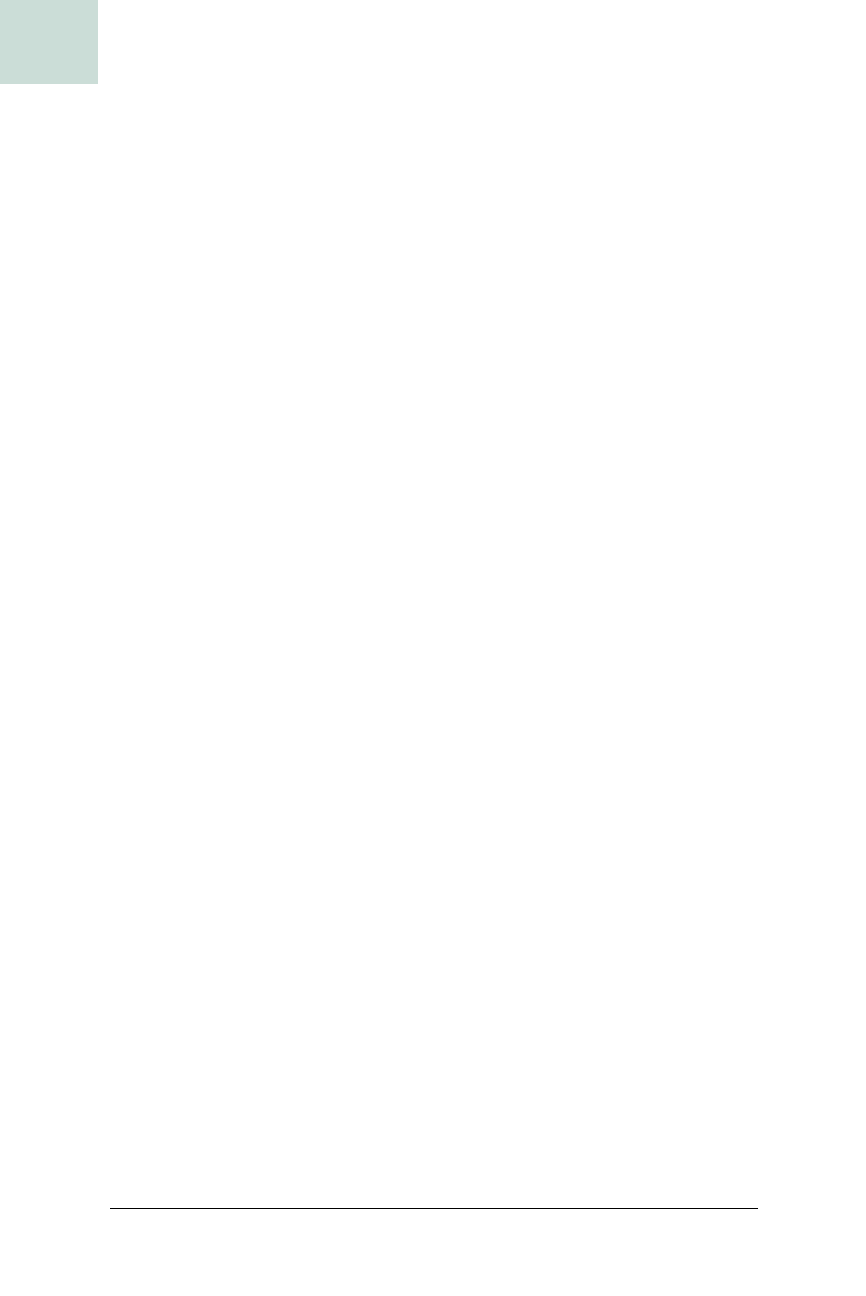
390
|
Chapter 10, Audio
#76 Play Non-Trivial Audio
HACK
Notice in the constructor that, as with the Clip, the way to get an actual
Line object is to construct a DataLine.Info object and then pass that to
AudioSystem
. This time, you construct a
DataLine.Info
class with both the
SourceDataLine
class—you need this subclass of
Line
because it provides the
write( )
method with which you supply bytes to the
Line
—and an
AudioFormat
object describing the data you’ll be supplying. Assuming that
doesn’t throw a
LineUnavailableException (and it shouldn’t, because the
format is already known to be PCM, which JavaSound always supports),
you’ll have a line that you can open and start writing bytes to.
As mentioned previously, the key issue for the thread that reads bytes from
the file and writes them to the
Line is that it has to be aware of frame bound-
aries. In this code,
readPoint indicates the index of the buffer to start read-
ing bytes into. When you have an incomplete frame after reading from the
input stream, you copy the bytes from the incomplete frame to the front of
the buffer in preparation for the next read. For example, if you have a frame
size of 4, and
bytesRead % 4 equals 3, then you copy those 3 bytes to the front
of the buffer and set
readPoint to 3. The next read( ) will start at 3, and the
first byte read into the buffer will complete the frame from the previous
read( ).
Big Files, Big Sound
Since this is still in the realm of JavaSound, much of what was shown in the
Clip-based hack still works. A demo application simply has to provide
PCMFilePlayer with a file and then start it. Since PCMFilePlayer exposes its
Line through a get method, you can even wire up as a LineListener and get
notified of
STOP, START, OPEN, and CLOSE LineEvents. Example 10-9 shows the
simple GUI, using
PCMFilePlayer.
Example 10-9. Playing arbitrarily long uncompressed WAV or AIFF audio
import javax.sound.sampled.*;
public class StreamingLineSound extends Object
implements LineListener {
File soundFile;
JDialog playingDialog;
PCMFilePlayer player;
public static void main (String[] args) {
JFileChooser chooser = new JFileChooser( );
chooser.showOpenDialog(null);
File f = chooser.getSelectedFile( );
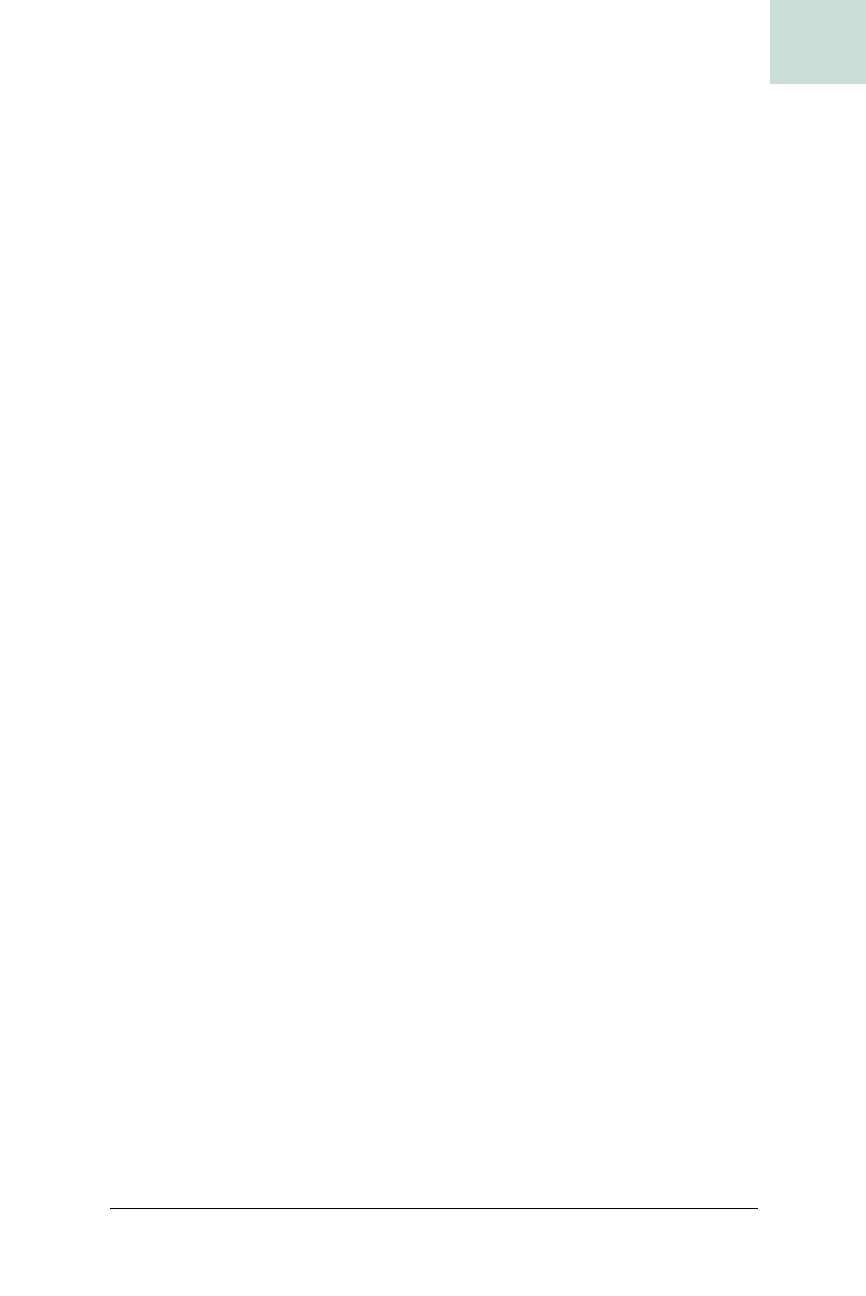
Play Non-Trivial Audio #76
Chapter 10, Audio
|
391
HACK
When run, this class shows a dialog box (seen in Figure 10-7), identical to
the one produced in “Play a Sound with JavaSound”
[Hack #71]. The only dif-
ference is that this one can stay up potentially indefinitely, since the player
can keep reading and writing bytes forever.
try {
StreamingLineSound s = new StreamingLineSound (f);
} catch (Exception e) {
e.printStackTrace( );
}
}
public StreamingLineSound (File f)
throws LineUnavailableException, IOException,
UnsupportedAudioFileException {
soundFile = f;
// prepare a dialog to display while playing
JOptionPane pane = new JOptionPane ("Playing " + f.getName( ),
JOptionPane.PLAIN_MESSAGE);
playingDialog = pane.createDialog (null, "Streaming Sound");
playingDialog.pack( );
player = new PCMFilePlayer (soundFile);
player.getLine( ).addLineListener (this);
player.start( );
}
// LineListener
public void update (LineEvent le) {
LineEvent.Type type = le.getType( );
if (type == LineEvent.Type.OPEN) {
System.out.println ("OPEN");
} else if (type == LineEvent.Type.CLOSE) {
System.out.println ("CLOSE");
System.exit (0);
} else if (type == LineEvent.Type.START) {
System.out.println ("START");
playingDialog.setVisible(true);
} else if (type == LineEvent.Type.STOP) {
System.out.println ("STOP");
playingDialog.setVisible(false);
player.line.close( );
}
}
}
Example 10-9. Playing arbitrarily long uncompressed WAV or AIFF audio (continued)
Get Swing Hacks now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.