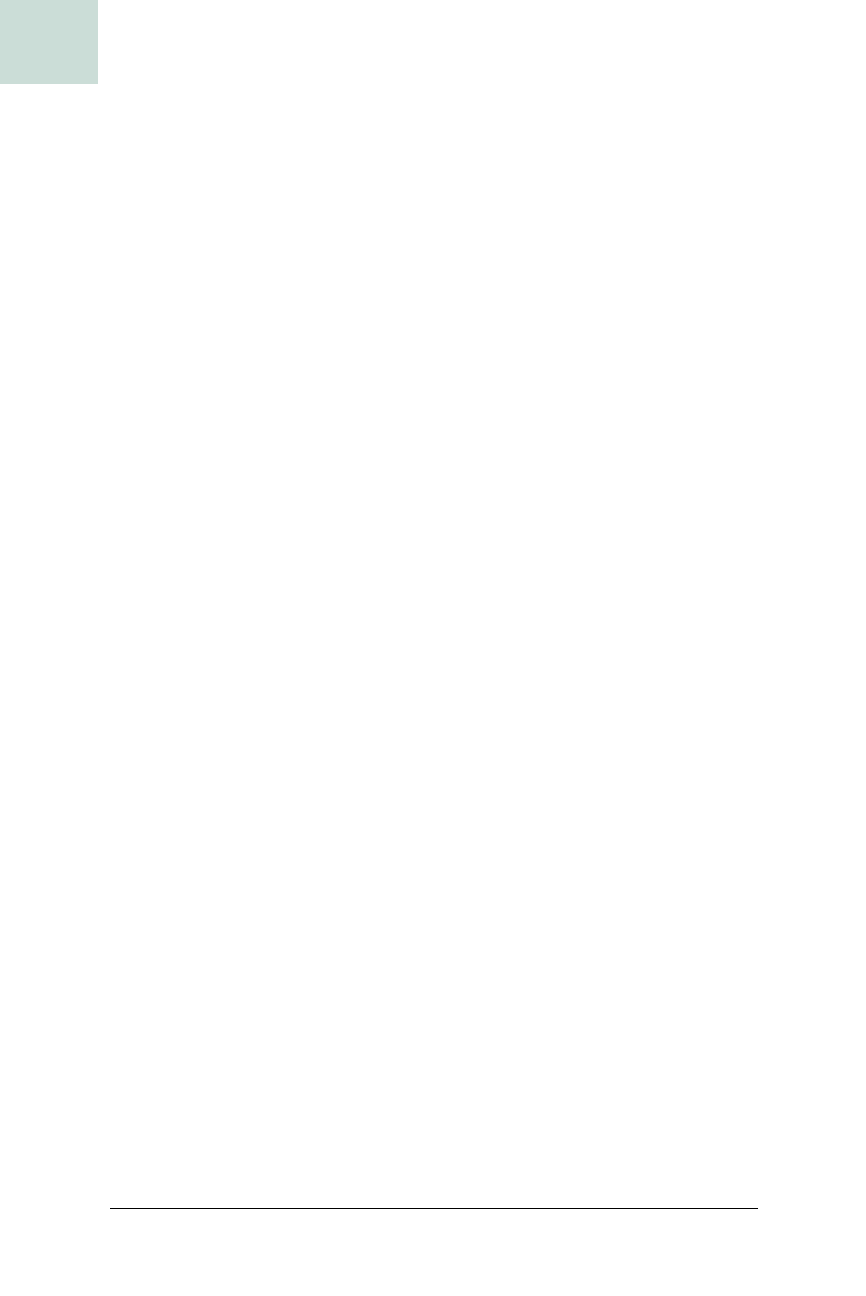
344
|
Chapter 9, Drag-and-Drop
#67 Handle Dropped Images
HACK
drop( ) asks the Transferable for supported DataFlavors in the order you’d
prefer to deal with them. First, obviously, is
DataFlavor.imageFlavor. If this
is supported, you can trivially cast the data object returned by
Transferable.
getTransferData( )
to an Image.
The next easiest thing to deal with is the Java file list, denoted by the MIME
type
application/x-java-file-list, and handled by the DataFlavor constant
javaFileListFlavor. Given Swing’s support for various image file formats,
you can cast the transfer data to a
java.util.List, iterate over its members,
cast each one to a
File, and make an ImageIcon from each file’s path. URLs
work pretty much the same way: cast the transfer data to a
java.net.URL and
make an
ImageIcon from it.
URI lists take just a little work on your part. RFC 2483 defines these as
being some number of URIs, separated by CRLF pairs. It’s trivial to take the
URI list as a string and send it to a
StringTokenizer to pick out each URI,
which can then be passed to the
ImageIcon constructor.
Shut Up and Drag
Start up the demo and drag over an image from your favorite application, as
shown in Figure 9-4.
// url-lists are defined by rfc 2483 as crlf-delimited
StringTokenizer izer = new StringTokenizer (uris, "\r\n");
while (izer.hasMoreTokens ( )) {
String uri = izer.nextToken( );
System.out.println (uri);
ImageIcon icon = new ImageIcon (uri);
showImageInNewFrame (icon);
}
gotData = true;
} else if (trans.isDataFlavorSupported (urlFlavor)) {
System.out.println ("url flavor is supported");
URL url = (URL) trans.getTransferData (urlFlavor);
System.out.println (url.toString( ));
ImageIcon icon = new ImageIcon (url);
showImageInNewFrame (icon);
gotData = true;
}
} catch (Exception e) {
e.printStackTrace( );
} finally {
System.out.println ("gotData is " + gotData);
dtde.dropComplete (gotData);
}
}
Example 9-5. Handling the image drop (continued)
Get Swing Hacks now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.