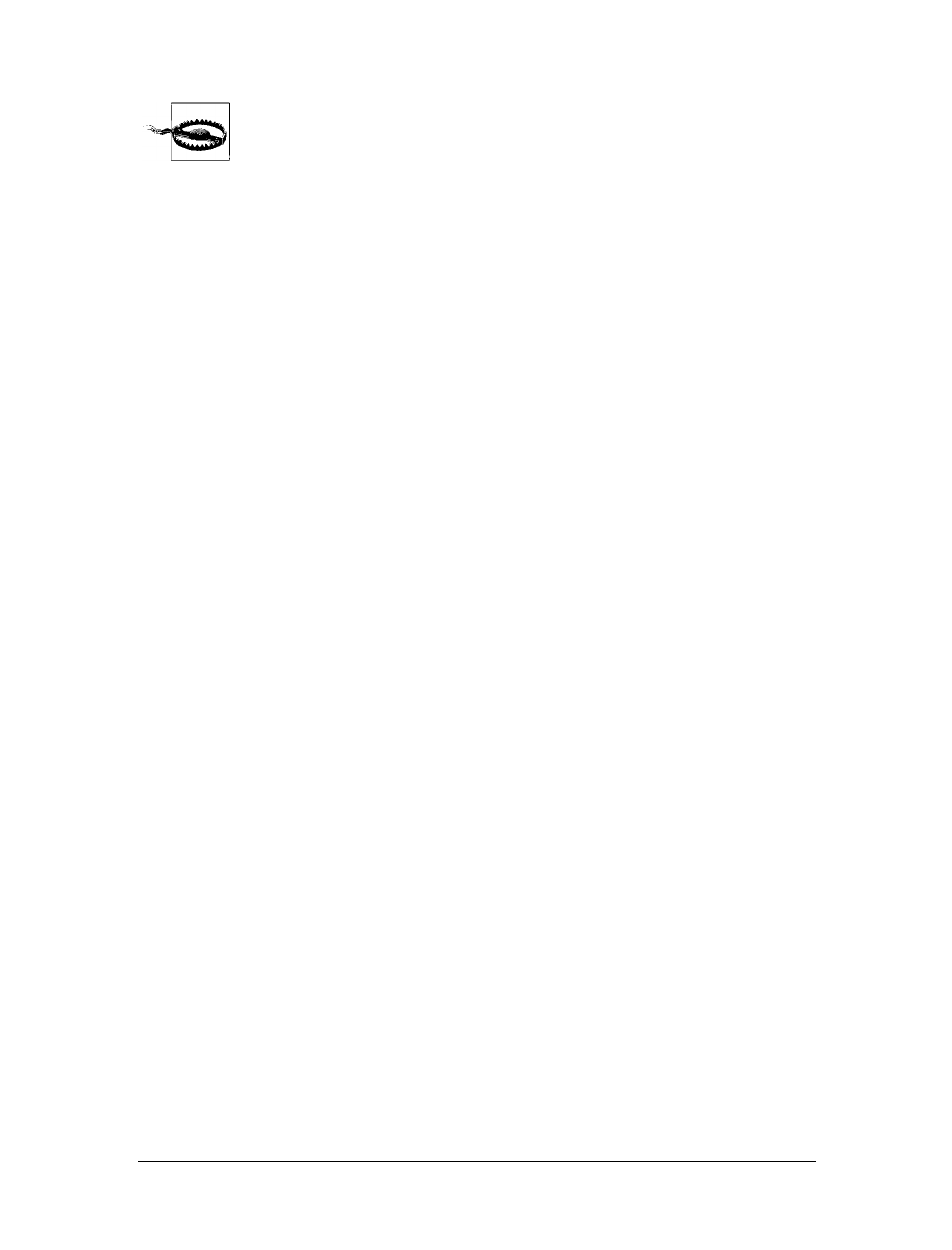
This is the Title of the Book, eMatter Edition
Copyright © 2007 O’Reilly & Associates, Inc. All rights reserved.
96
|
Chapter 3: Managing Connections
Remove the ActionScript to include NetDebug.as in your final movie
after you no longer need the debugging features. It adds unnecessary
file size to the published .swf.
Subclassing the NetConnection Class
You can extend the features of the NetConnection class by creating a subclass based
on it. Creating subclasses lets you encapsulate code that operates on the NetConnec-
tion object within the subclass’s methods such as connect( ), onStatus( ),orclose( ).In
turn, this can lead to more modular and easier to maintain applications. The key to
doing this is to use the super operator. Example 3-8 shows a very simple example of
how to extend the NetConnection class and then how to use it within a simple test
script.
Example 3-8 uses AS 1.0 syntax so it works in both Flash MX and Flash MX 2004.
This also allows you to compile using ActionScript 1.0 under File
➝ Publish Settings ➝
ActionScript. Example 3-10 provides an alternative implementation that uses AS 2.0
syntax and the v2 UI component’s EventDispatcher class.
Example 3-8. A simple extension of the NetConnection class
// Constructor function must call super( ).
function NetConnectionSubClass ( ) {
super( );
}
// Subclass NetConnection by assigning an instance of it
// to the prototype object of the new subclass.
NetConnectionSubClass.prototype = new NetConnection( );
// A simple demonstration onStatus( ) event handler that just
// writes out each info.code message.
NetConnectionSubClass.prototype.onStatus = function (info) {
trace("info.code: " + info.code);
};
/* This connect( ) method may be passed any number of arguments.
* The apply( ) method makes sure all arguments are passed into
* the super.connect( ) method when it is called.
*/
NetConnectionSubClass.prototype.connect = function ( ) {
return super.connect.apply(super, arguments);
};
// Create an instance of the NetConnectionSubClass subclass.
lobby_nc = new NetConnectionSubClass( );
// Attempt the connection.
if (lobby_nc.connect("rtmp:/testLobby/", "Guest", "Guest")) {
trace("Attempting connection...");
}
else {
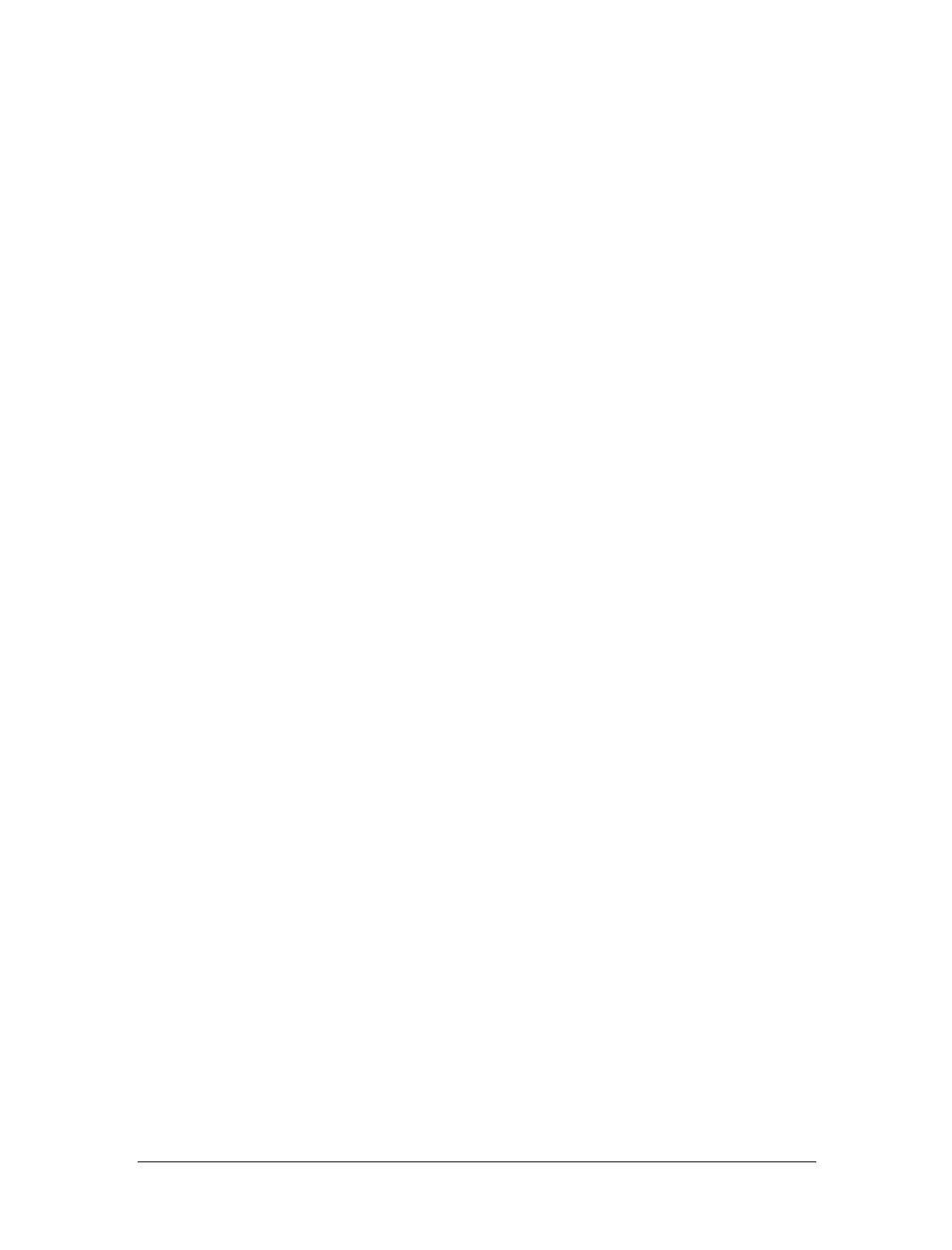
This is the Title of the Book, eMatter Edition
Copyright © 2007 O’Reilly & Associates, Inc. All rights reserved.
Subclassing the NetConnection Class
|
97
The super operator is used in two different ways in Example 3-8. First, it must be
called within the constructor function as follows:
super( );
Invoking super( ) in this way calls the NetConnection constructor (the superclass’s
constructor). Second, whenever a NetConnection method must be called by a sub-
class’s method, the super operator can be used to call the superclass’s method. In this
example, when the NetConnectionSubClass.connect( ) method is called, it in turn uses
super to call the NetConnection.connect( ) method as follows:
return super.connect.apply(super, arguments);
Using the apply( ) method provides a good, general purpose way to call the super-
class’s connect( ) method. Passing
super as a parameter ensures that connect( ) is
called as a method of the NetConnection superclass and passing
arguments insures
that all the parameters passed to the subclass’s connect( ) method are passed on to
the superclass’s connect( ) method.
A simpler, but less general purpose, way to call the NetConnection.connect( ) method
is to specifically pass individual parameters this way:
NetConnectionSubClass.prototype.connect = function (targetURI, userName, password) {
return super.connect(targetURI, userName, password);
};
In cases in which parameter values must be manipulated before being passed to the
superclass’s connect( ) method or those in which not all parameters must be passed
on, it is often simpler to avoid using the apply( ) method.
On its own, Example 3-8 shows the basic mechanics of creating a subclass of the Net-
Connection class, but let’s see what advantages this offers. Looking back at
Example 3-4 and Example 3-6, you will see that before calling the connect( ) or close( )
methods, the
handleCloseEvents flag had to be set each time. This is extra work that
shouldn’t have to be done in the doConnect( ), doChat( ), and doLobby( ) functions.
In fact, these details can be hidden inside a NetConnection subclass’s methods as fol-
lows:
// close( ) turns off the handleCloseEvents flag before closing the connection.
LobbyChatConnection.prototype.close = function ( ) {
this.handleCloseEvents = false;
super.close( );
};
If we modify the connect( ) method to set the handleCloseEvents flag as well, the
doLobby( ) function can be greatly simplified:
// Called when the Lobby button in a chat room is clicked.
function doLobby(btn) {
trace("Can't attempt connection. Is the URI correct?");
}
Example 3-8. A simple extension of the NetConnection class (continued)
Get Programming Flash Communication Server now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.