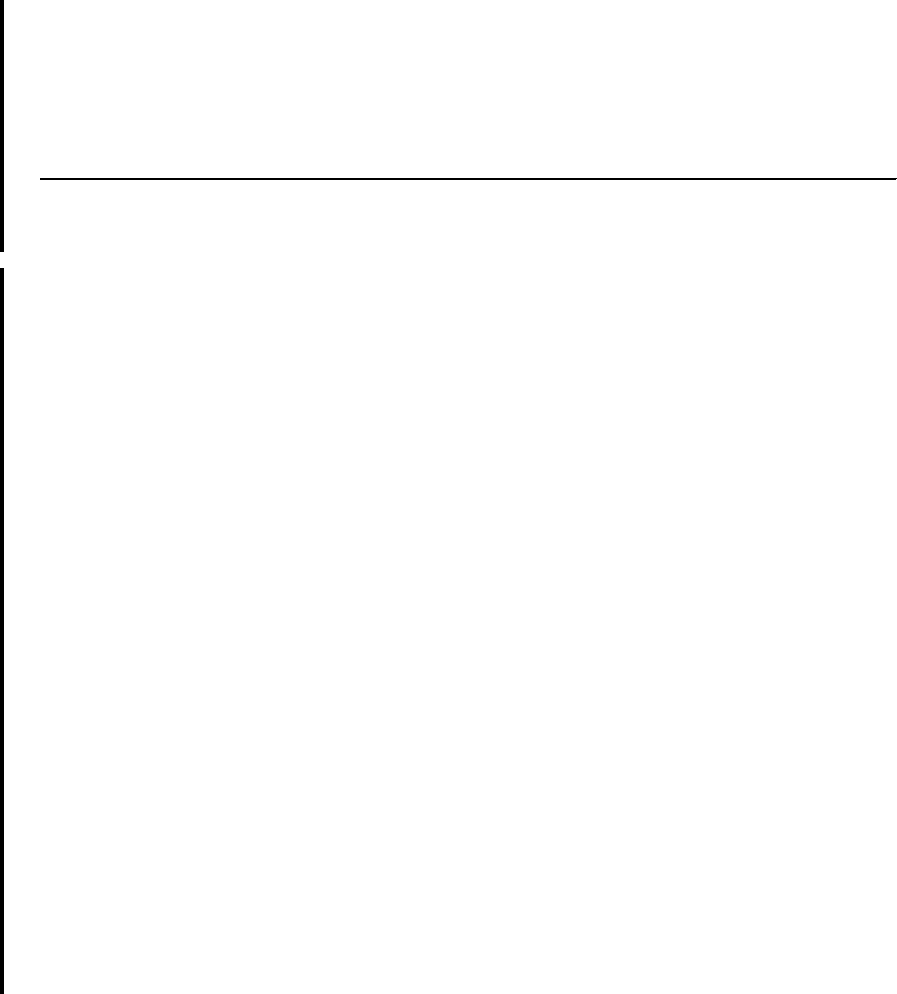
328 Patterns: Implementing Self-Service in an SOA Environment
}
catch (Exception e) {
e.printStackTrace();
throw new MessageContextException();
}
return true;
}
}
10.6.2 Create the Aggregator mediation
This mediation is associated with the reply queue destination. When an incoming
message has the delivery type All, the request is sent to both the HomeDelivery
and the BusinessDelivery services. The reverse routing path is modified to new
reply queue destination. The response from both the messages is sent to this
reply queue destination where the Aggregator mediation is invoked. The
mediation aggregates the responses from both services into one response and
sends it back to the client.
The following are the steps to create the aggregator mediation:
1. Build the code:
a. Create a simple project called Aggregator.
b. Right-click the Aggregator project and select Add → Others → Java
Class. Name the class AggregatorMediation.
c. Implement the MediationHandler interface and add the code required for
aggregating the responses in the handle method (see “Aggregator code”
on page 329).
d. Create a JAR file from the package and call it Aggregator.jar.
2. Create the mediation EJB:
a. Create an EJB project called AggregatorEJB with an EAR file name of
AggregatorEJBEAR.
b. Drag and drop Aggregator.jar to the Utility JARS folder in
AggregatorEJBEAR.
3. Define the mediation handler in the EJB deployment descriptor:
a. Expand the AggregatorEJB project in the navigator and double-click
Deployment Descriptor.
b. Select the Mediation Handler tab.
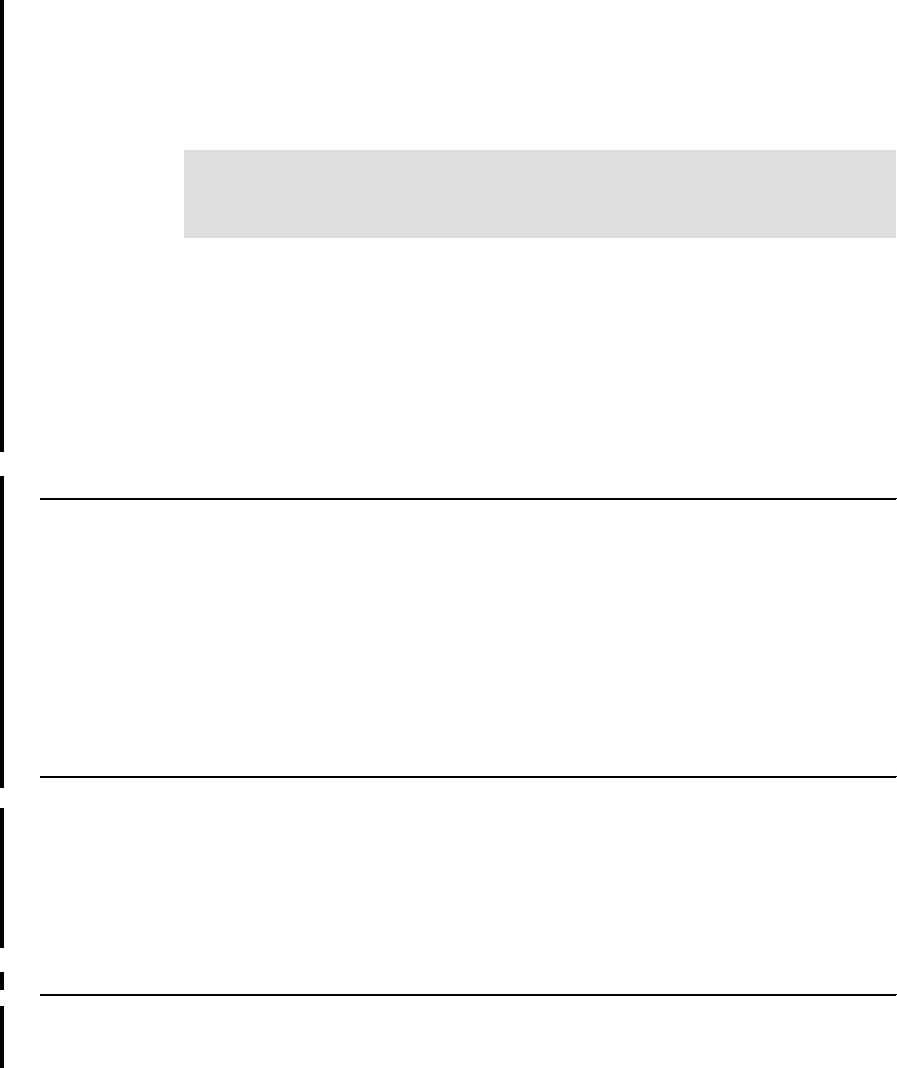
Chapter 10. Web services scenario 329
c. Click Add and enter AggregatorHandler as the name. Enter
myPackage.AggregatorMediation as the handler class.
d. Save the descriptor.
Aggregator code
The handle() method (Example 10-8) is responsible for the message handling
and aggregation of the response messages, and sending the aggregated
response back to the original requester. The handle() method is invoked by the
arrival of a message on the queue that it has been configured to mediate,
DeliveryResponseDestination.
The handle() method casts the message context to an SIMessageContext and
retrieves the forward routing path for the message.
Example 10-8 AggregatorMediation code part 1
public class AggregatorMediation implements MediationHandler {
public boolean handle(MessageContext context) throws MessageContextException {
SIMessageContext ctx =(SIMessageContext)context;
String busName = ctx.getSession().getBusName();
SIMessage message = ctx.getSIMessage();
List frp = message.getForwardRoutingPath();
SIMediationSession session = ctx.getSession();
SIMessage otherMessage=null;
SIDestinationAddressFactory factory =
SIDestinationAddressFactory.getInstance();
Next (Example 10-9), it retrieves the name of a temporary storage queue it will
use to hold received messages. The code checks to see if it can receive a
message from the temporary queue. If no message is there, then the message
that has arrived on the mediated queue is the first response. If it is the first
response, the forward routing path is set to the temporary queue and the
message is stored there.
Example 10-9 AggregatorMediation code part 2
try {
otherMessage = session.receive((String)context.getProperty("TempDataDestination"));
if (otherMessage == null)
Note: The entire source code for the aggregator mediation can be seen by
downloading the sample application. The code is in the Aggregator.jar file in
the AggregatorEJB project.
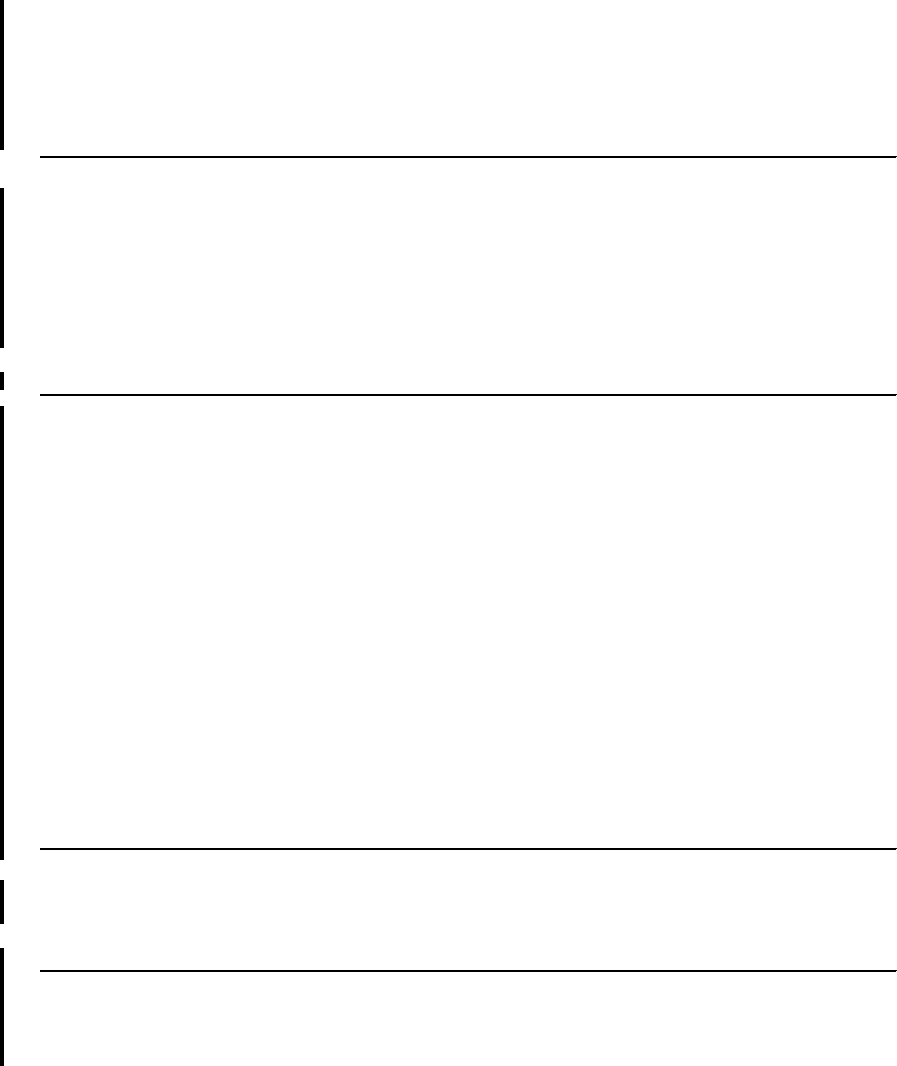
330 Patterns: Implementing Self-Service in an SOA Environment
{
SIDestinationAddress queue= factory.
createSIDestinationAddress((String)context.getProperty("TempDataDestination"),busName);
frp.clear();
frp.add(queue);
message.setForwardRoutingPath(frp);
}
If there is a message on the temporary queue, this response is the second
message (Example 10-10).
The first message (otherMessage) is retrieved from the temporary queue and the
account number information (getAccountReturn) is extracted. Next, the account
information is extracted from the second message (message). Then the two
responses are combined and written back into the current (second) message.
Example 10-10 AggregatorMediation code part 3
else
{
DataGraph graph = null;
try {
graph = otherMessage.getDataGraph();
DataObject rootNode = graph.getRootObject();
DataObject infoNode = rootNode.getDataObject("Info");
DataObject bodyNode = infoNode.getDataObject("body");
DataObject part1Node = bodyNode.getDataObject("parameters");
String ticker = part1Node.getString("getAccountReturn");
DataGraph graph1 = message.getDataGraph();
DataObject rootNode1 = graph1.getRootObject();
DataObject infoNode1 = rootNode1.getDataObject("Info");
DataObject bodyNode1 = infoNode1.getDataObject("body");
DataObject part1Node1 = bodyNode1.getDataObject("parameters");
String ticker1 = part1Node1.getString("getAccountReturn");
part1Node1.setString("getAccountReturn",
ticker+","+ticker1);
Lastly (Example 10-11), the forward routing path is changed so the response will
be sent back to the client.
Example 10-11 AggregatorMediation code part 4
String newDestination =
(String)context.getProperty("InboundServiceClientResponseDest");
SIDestinationAddress newAddress = factory.
Get Patterns: Implementing Self-Service in an SOA Environment now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.