2
Introduction to OpenCV 2.x
Include files
After installing the OpenCV library and setting up our programming environment, our next task is to make
something interesting happen with code. In order to do this, we’ll have to discuss header files. Fortunately,
the headers reflect the new, modular structure of OpenCV introduced in Chapter 1. The main header file of
interest is …/include/opencv2/opencv.hpp. This header file just calls the header files for each OpenCV
module:
#include "opencv2/core/core_c.h"
Old C data structures and arithmetic routines.
#include "opencv2/core/core.hpp"
New C++ data structures and arithmetic routines.
#include "opencv2/flann/miniflann.hpp"
Approximate nearest neighbor matching functions. (Mostly for internal use)
#include "opencv2/imgproc/imgproc_c.h"
Old C image processing functions.
#include "opencv2/imgproc/imgproc.hpp"
New C++ image processing functions.
#include "opencv2/video/photo.hpp"
Algorithms specific to handling and restoring photographs.
#include "opencv2/video/video.hpp"
Video tracking and background segmentation routines.
#include "opencv2/features2d/features2d.hpp"
Two-dimensional feature tracking support.
#include "opencv2/objdetect/objdetect.hpp"
Cascade face detector; latent SVM; HoG; planar patch detector.
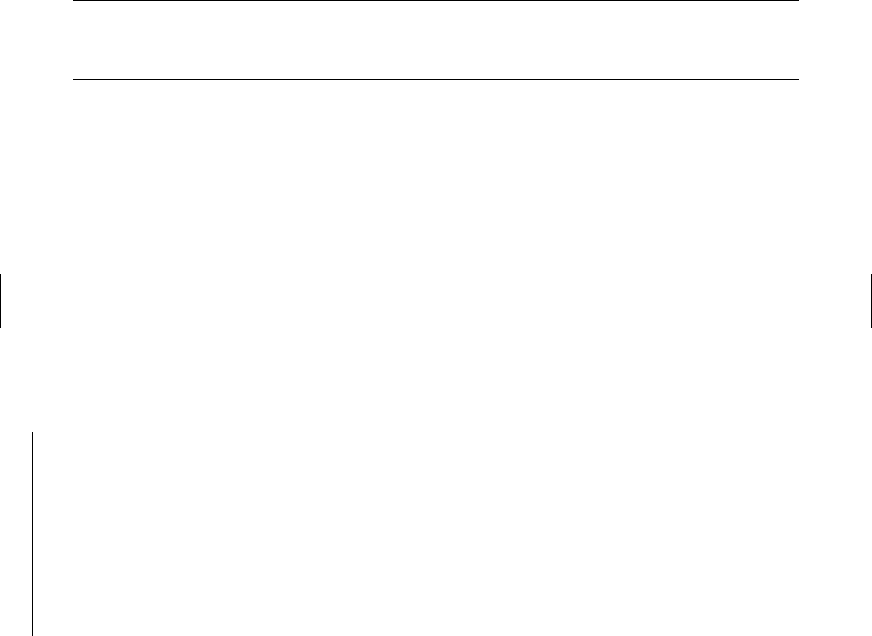
#include "opencv2/calib3d/calib3d.hpp"
Calibration and stereo.
#include "opencv2/ml/ml.hpp"
Machine learning: clustering, pattern recognition.
#include "opencv2/highgui/highgui_c.h"
Old C image display, sliders, mouse interaction, I/O.
#include "opencv2/highgui/highgui.hpp"
New C++ image display, sliders, buttons, mouse, I/O.
#include "opencv2/contrib/contrib.hpp"
User-contributed code: flesh detection, fuzzy mean-shift tracking, spin images, self-similar features.
You may use the include file opencv.hpp to include any and every possible OpenCV function but, since it
includes everything, it will cause compile time to be slower. If you are only using, say, image processing
functions, compile time will be faster if you only include opencv2/imgproc/imgproc.hpp. These include
files are located on disk under the …/modules directory. For example, imgproc.hpp is located at
…/modules/imgproc/include/opencv2/imgproc/imgproc.hpp. Similarly, the sources for the functions
themselves are located under their corresponding src directory. For example, cv::Canny() in the
imgproc module is located in …/modules/improc/src/canny.cpp.
With the above include files, we can start our first C++ OpenCV program.
Legacy code such as the older blob tracking, hmm face detection, condensation tracker,
and eigen objects can be included using opencv2/legacy/legacy.hpp, which is located in
…/modules/legacy/include/opencv2/legacy/legacy.hpp.
First Program—Display a Picture
OpenCV provides utilities for reading from a wide array of image file types, as well as from video and
cameras. These utilities are part of a toolkit called HighGUI, which is included in the OpenCV package. On
the http://opencv.org site, you can go to the tutorial pages off of the documentation links at
http://docs.opencv.org/doc/tutorials/tutorials.html to see tutorials on various aspects of using OpenCV.
In the tutorial section, the “introduction to OpenCV” tutorial explains how to set up OpenCV for
various combinations of operating systems and development tools.
We will use an example from the “highgui module” to create a simple program that opens an image and
displays it on the screen (Example 2-1).
Example 2-1: A simple OpenCV program that loads an image from disk and displays it on the screen
#include <opencv2/opencv.hpp> //Include file for every supported OpenCV function
int main( int argc, char** argv ) {
cv::Mat img = cv::imread(argv[1],-1);
if( img.empty() ) return -1;
cv::namedWindow( "Example1", cv::WINDOW_AUTOSIZE );
cv::imshow( "Example1", img );
cv::waitKey( 0 );
cv::destroyWindow( "Example1" );
}
Note that OpenCV functions live within a namespace called cv. To call OpenCV functions, you must
explicitly tell the compiler that you are talking about the cv namespace by prepending cv:: to each function
Get Learning OpenCV, 2nd Edition now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.