Name
SynchronousQueue<E>
Synopsis
This
BlockingQueue
implementation is the degenerate case of a bounded queue with a
capacity of zero. Every call to put( )
blocks
until a corresponding call to take( )
, and vice
versa. You can think of this as an Exchanger
that
does only a one-way exchange.
The size( )
and remainingCapacity(
)
methods always return 0. The peek( )
method always returns null
. The iterator(
)
method returns an Iterator
for which
the hasNext( )
method returns
false
.
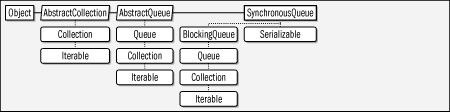
Figure 16-93. java.util.concurrent.SynchronousQueue<E>
public class SynchronousQueue<E> extends java.util.AbstractQueue<E> implements BlockingQueue<E>, Serializable { // Public Constructors public SynchronousQueue( ); public SynchronousQueue(boolean fair); // Methods Implementing BlockingQueue public int drainTo(java.util.Collection<? super E> c); public int drainTo(java.util.Collection<? super E> c, int maxElements); public boolean offer(E o); public boolean offer(E o, long timeout, TimeUnit unit) throws InterruptedException; public E poll(long timeout, TimeUnit unit) throws InterruptedException; public void put(E o) throws InterruptedException; public int remainingCapacity( ); constant public E take( ) throws InterruptedException; // Methods Implementing Collection public void clear( ); empty public boolean contains(Object o); constant public boolean containsAll(java.util.Collection<?> c); public boolean ...
Get Java in a Nutshell, 5th Edition now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.