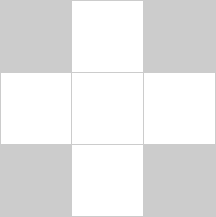
i
i
i
i
i
i
i
i
19
Kelvin Sung
Building Interactive Graphics
Applications
While most of the other chapters in this book discuss the fundamental algorithms
in the field of computer graphics, this chapter treats the integration of these al-
gorithms into applications. This is an important topic since the knowledge of
fundamental graphics algorithms does not always easily lead to an understanding
of the best practices in implementing these algorithms in real applications.
We start with a simple example: a program that allows the user to simulate the
shooting of a ball (under the influence of gravity). The user can specify initial ve-
locity, create balls of different sizes, shoot the ball, and examine the parabolic free
fall of the ball. Some fundamental concepts we will need include mesh structure
for the representation of the ball (sphere); texture mapping, lighting, and shading
for the aesthetic appearance of the ball; transformations for the trajectories of the
ball; and rasterization techniques for the generation of the images of the balls.
To implement the simple ball shooting program, one also needs knowledge of
• graphical user interface (GUI) systems for efficient and effective user inter-
action;
• software architecture and design patterns for crafting an implementation
framework that is easy to maintain and expand;
• application program interfaces (APIs) for choosing the appropriate support
and avoiding a massive amount of unnecessary coding.
467

i
i
i
i
i
i
i
i
468 19. Building Interactive Graphics Applications
To gain an appreciation for these three important aspects of building the ap-
plication, we will complete the following steps:
• analyze interactive applications;
• understand different programming models and recognize important func-
tional components in these models;
• define the interaction of the components;
• design solution frameworks for integrating the components; and
• demonstrate example implementations based on different sets of existing
APIs.
We will use the ball shooting program as our example and begin by refining the
detailed specifications. For clarity, we avoid graphics-specific complexities in
3D space and confine our example to 2D space. Obviously, our simple program
is neither sophisticated nor representative of real applications. However, with
slightly refined specifications, this example contains all the essential components
and behavioral characteristics of more complex real-world interactive systems.
We will continue to build complexity into our simple example, adding new
concepts until we arrive at a software architecture framework that is suitable for
building general interactive graphics applications. We will examine the validity of
our results and discuss how the lessons learned from this simple example can be
applied to other familiar real-world applications (e.g., PowerPoint, Maya, etc.).
19.1 The Ball Shooting Program
Our simple program has the following elements and behaviors.
• The balls (objects). The user can left-mouse-button-click and drag-out a
new ball (circle) anywhere on the screen (see Figure 19.1). Dragging-out a
ball includes:
–(A).Initial mouse-button-click position defines the center of the cir-
cle;
–(B).Mouse button downand moving the mouse is the dragging action;
–(C).Current mouse position while dragging allows us to define the
radius and the initial velocity. The radius R (in pixel units) is the dis-
tance to the center defined in (A). The vector from the current position
to the center is the initial velocity V (in units of pixel per second).
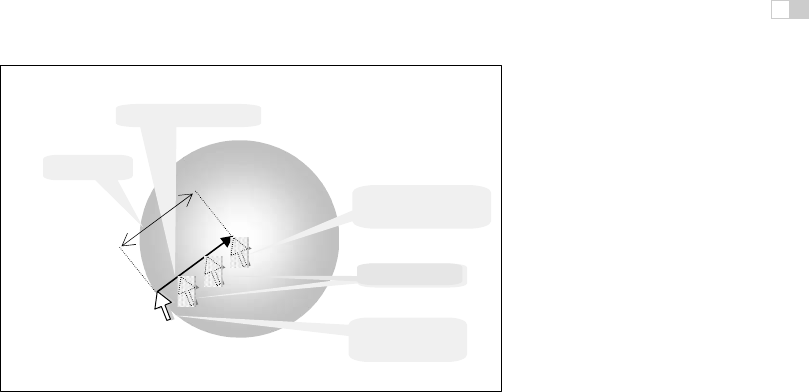
i
i
i
i
i
i
i
i
19.1. The Ball Shooting Program 469
(R): Radius
(V): Initial velocity
(A): Initial mouse
click position
Dragging
(C) Current
mouse position
(
B
)
Dra
gg
in
g
Figure 19.1. Dragging out a ball.
Once created, the ball will begin traveling with the defined initial velocity.
• HeroBall (Hero/active object). The user can also right-mouse-button-
click to select a ball to be the current HeroBall. The HeroBall’s velocity
can be controlled by the slider bars (discussed below) where its velocity
is displayed. (A newly created ball is by default the current HeroBall.)
A right-mouse-button-click on unoccupied space indicates that no current
HeroBall exists.
• Velocity slider bars (GUI elements). The user can monitor and control
two slider bars (x-andy-directions with magnitudes) to change the veloc-
ity of the HeroBall. When there is no HeroBall, the slider bar values are
undefined.
• The simulation.
– Ball traveling/collisions (object intrinsic behaviors). A ball knows
how to travel based on its current velocity and one ball can potentially
collide with another. For simplicity, we will assume all balls have
identical mass and all collisions are perfectly elastic.
– G ravity (external effects on objects). The velocity of a ball is con-
stantly changing due to the defined gravitational force.
– Status bar (application state echo). The user can monitor the ap-
plication state by examining the information in the status bar. In our
application, the number of balls currently on the screen is updated in
the status bar.
Get Fundamentals of Computer Graphics, 3rd Edition now with the O’Reilly learning platform.
O’Reilly members experience books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.